Functions
Bruno Munari. Design as Art. Editori Laterza 1966.
Functions have Functionality
A function is a means for action. It is our first step towards learning about algorithms - a formal set of instructions. Whereas variables store data, functions define what we do with that data. They are therefore part of the control structures in a program. We can define actions such as; calculating the average number, moving a shape, drawing a polygon, applying colour, plotting data points, sorting a list of words...Indeed, our imagination is our only limit.
Functions help us get specific tasks done. In other words, they help us to encapsulate a concept within a single structure or block of code. This helps us organise our ideas in a clear and logical manner and this becomes essential with more complex programs. You'll use functions all the time and the neat thing with this fundamental concept is that once you have written your function, you can re-use it as many times as you wish, throughout the program and indeed in other programs too!
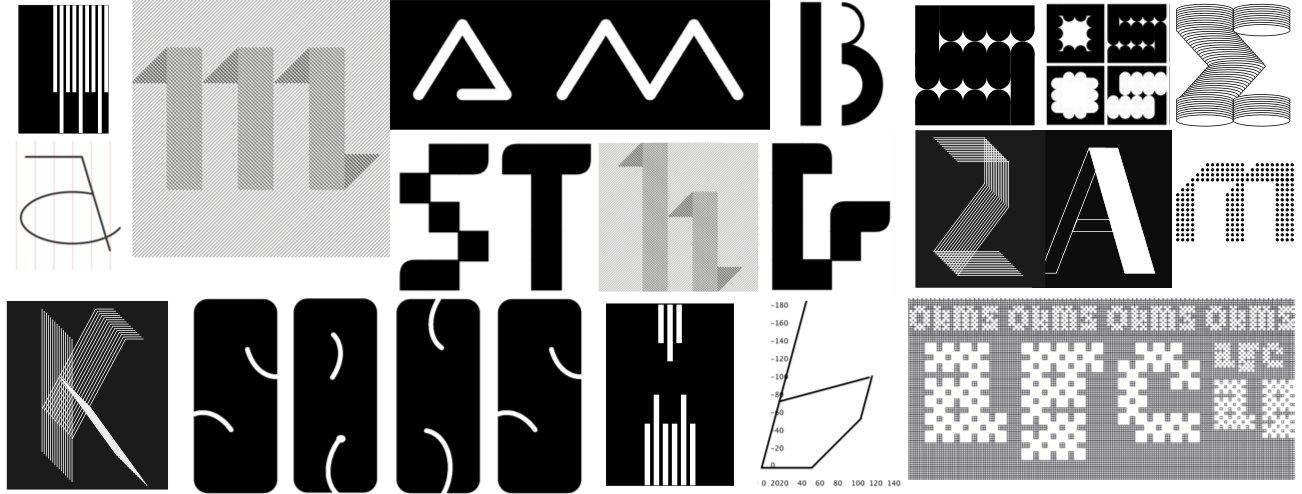
A mosaic of student work on modular type with functions.
There are two main steps to a function: 1). We declare a function, giving it a name and eventually its arguments. 2). We define our function within curly brackets - the body.
To declare a function we must give it a name, just as we did with a variable. We must also stipulate names for its parameters if our function receives arguments. Lets have a look at a simple example.
return type
‹name›
‹parameters›
‹body›
Eg 1. JavaScript
function
cross
( _size)
{
rect(0, 0, _size, _size/4);
rect(0, 0, _size, _size/4);
}
Eg 2. java
void
cross
(float _size)
{
rect(0, 0, _size, _size/4);
rect(0, 0, _size, _size/4);
}
Our function defines a shape - a cross - and if you look at the body of this function, you see that it is simply made up of two rectangles of different sizes. We could have used two lines to make our cross or something more fancy with vectors but I've made it simple. There are often more than one way to do things. Our function has one parameter named _size
and this is used in the body to designate the size of our shape. What this means then, is that when we call our function within the main part of our program, we can pass it a value and this value will determine the _size parameter and hence the size of our cross.
Eg 2. java
cross(20);
// a cross of 20 pixels
cross(120);
// a cross of 120 pixels
...
float crossSize = 75;
cross(crossSize);
// a cross of 75 pixels
Note that when we have a function that accepts arguments* we say that the value is passed into that function. It's important to grasp that concept of the passing in of values because one must understand the flow of data here. When we pass a value as an argument, that value becomes a parameter of our function. In our case, the parameter is defined as _size
. And because it is a parameter, we can equally pass into our function, yes you got it, a variable, as in the last example where we pass our variable crossSize
You are encouraged to explore these sketches. You'll notice that the basic cross function has more parameters in the examples on Observable. Try to understand what each parameter does and how it is connected within the main structure of the function. What new geometrical forms can you imagine by combining primitive shapes? Try to encapsulate that idea within a function. Write it down first on paper, think about the parameters, then write it in code. Experiment.
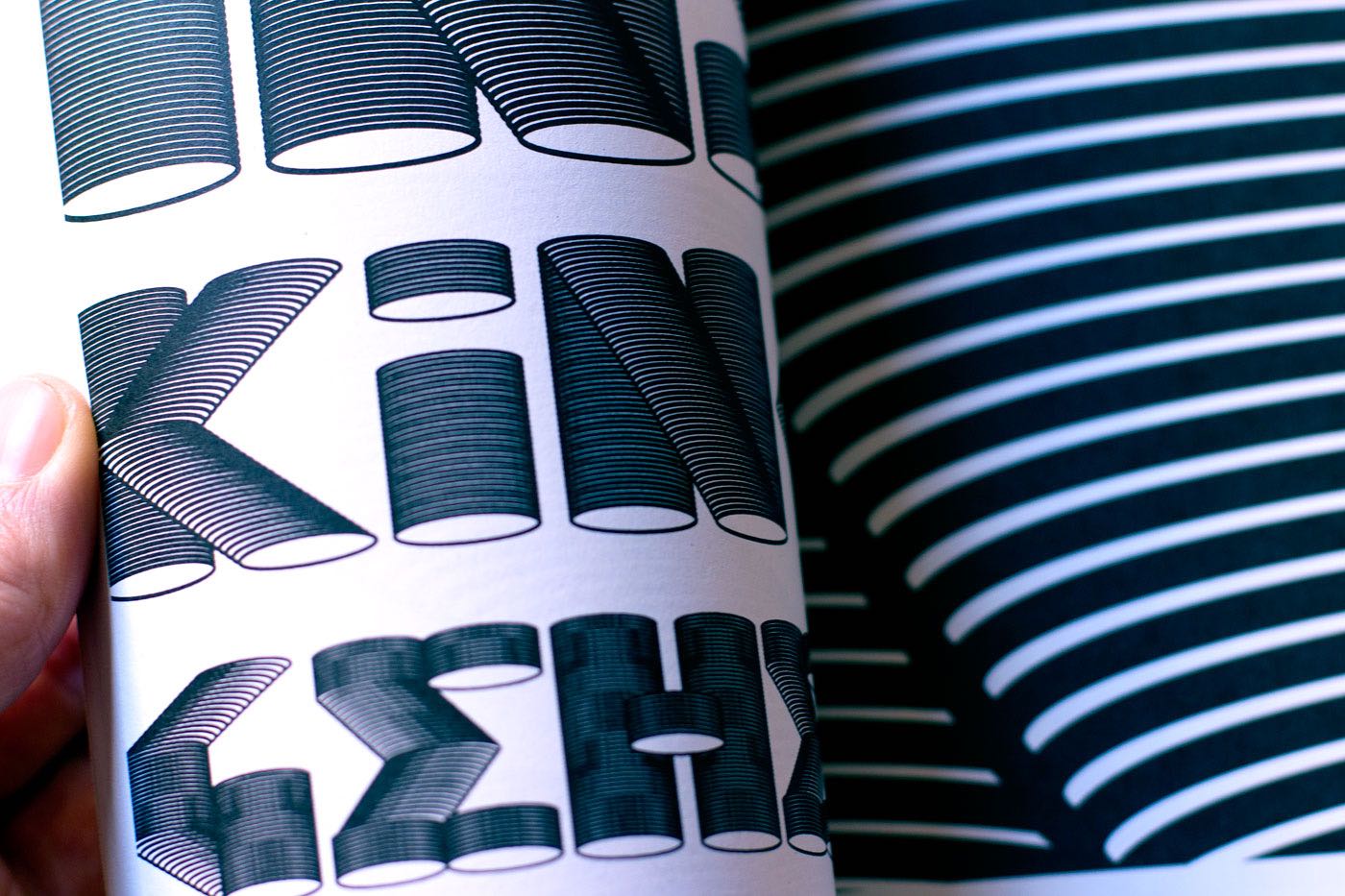
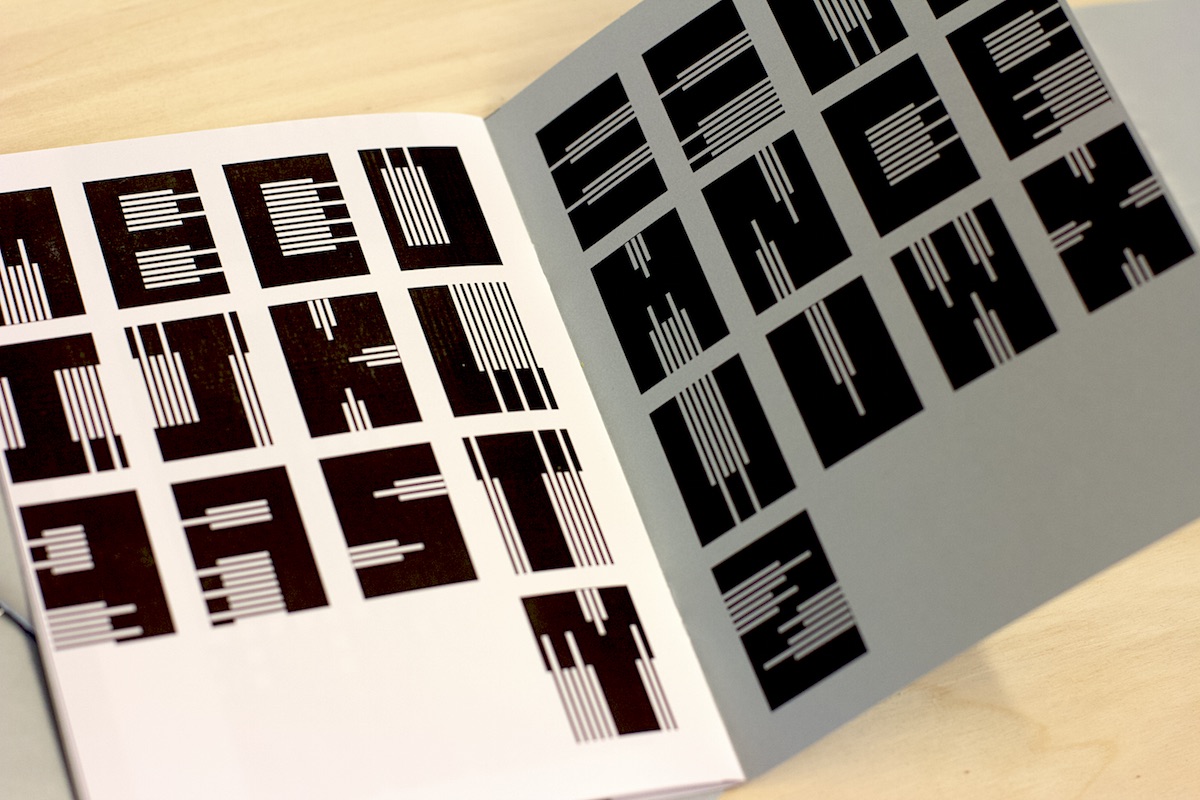
Typography exercise using functions. ESAD Amiens 2018.
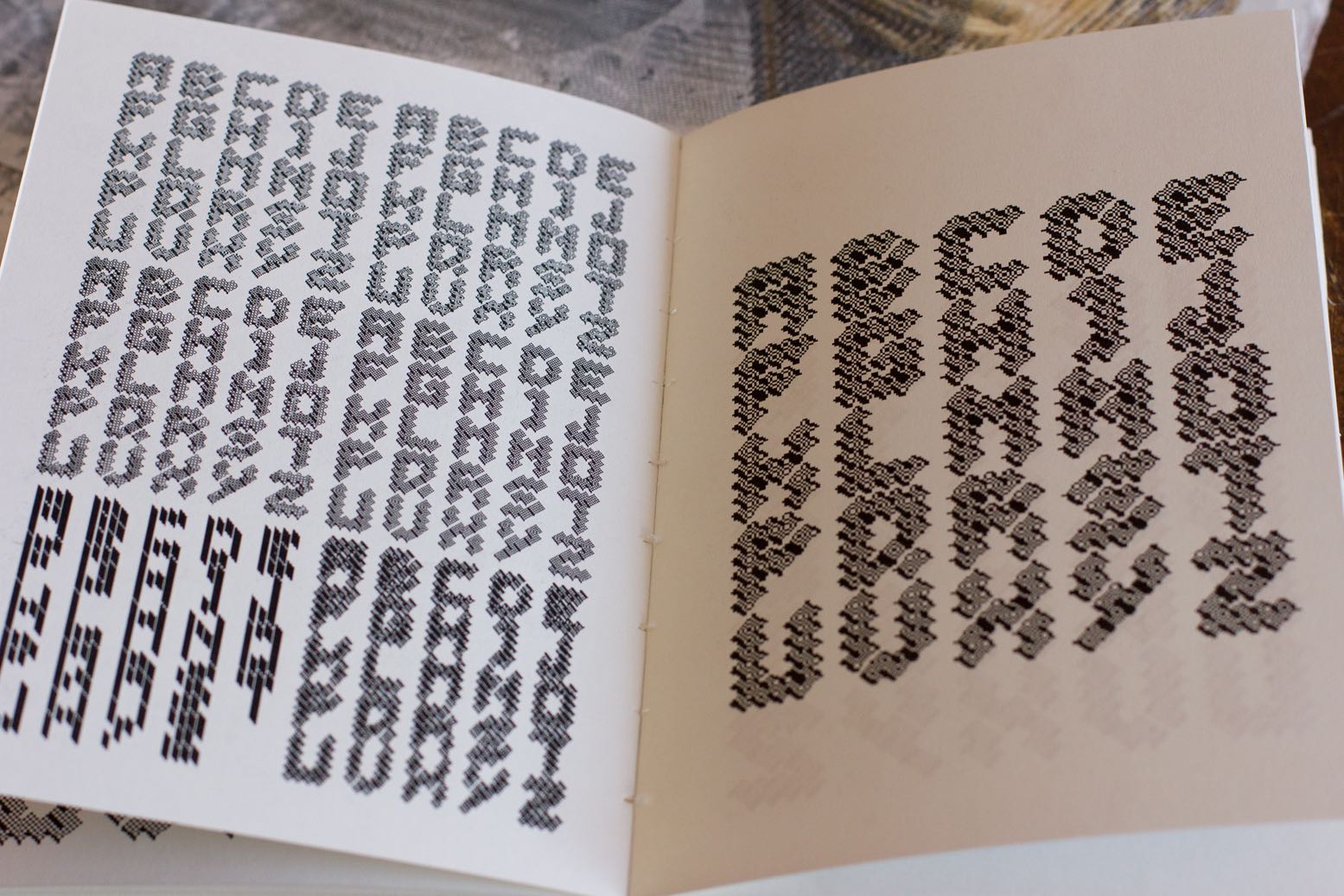
Typography exercise using functions. ESAD Amiens 2017.
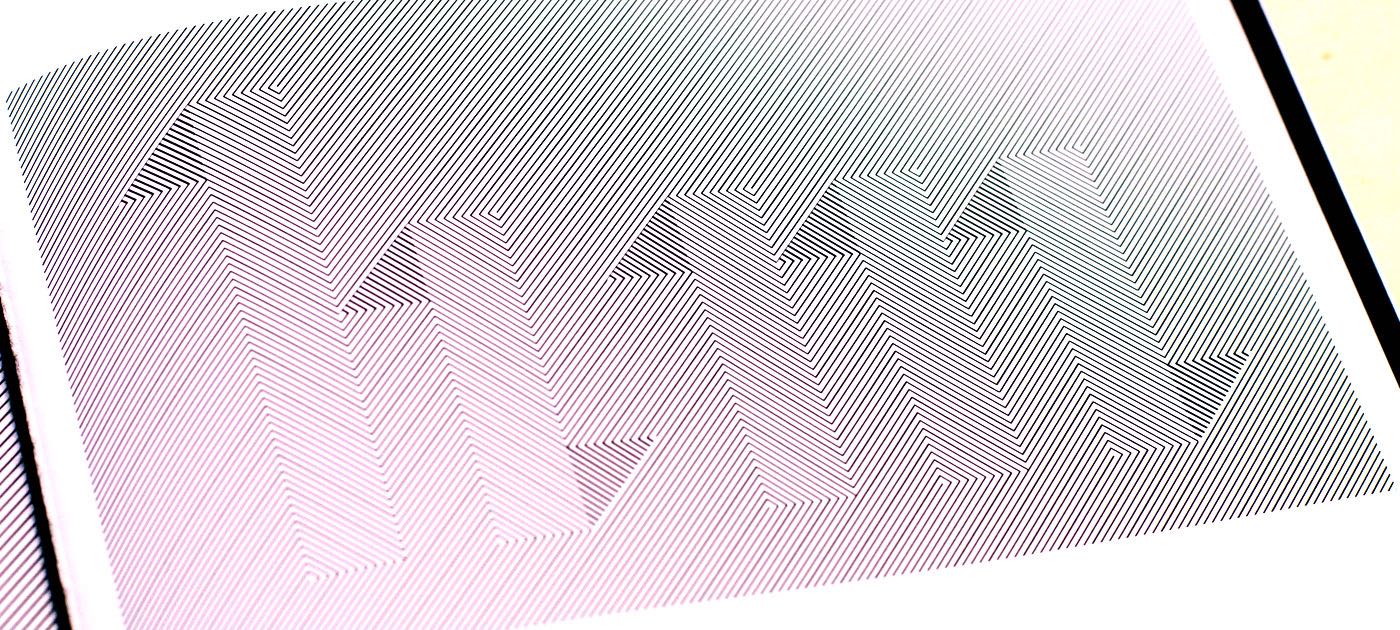
Typography exercise using functions. ESAD Amiens 2018.