Variables
Charles Babagge.
If we look back to the pioneering work of Charles Babbage and Ada Lovelace, we can trace the fundamental architecture of modern day computing; the concepts of data storage and control structures. Charles Babbage referred to these as the Store, where numbers are held and the Mill where arithmetic processing is performed. These were two distinct physical parts of the Analytical Engine, a machine that laid the foundations for both computing and programming. Up until the present day, little has changed in that architecture. Data is stored in memory (the store) and retrieved to apply operations on it (the mill).
All computers execute programs in this manner. When we write a computer program, we are essentially defining and organising data storage* and control structures. So, one holds the stuff - numbers, words, images, sound etc. - and the other deals with processing it. This is a really important piece of information to grasp. It will help you not only in writing programs but also in analysing and understanding programs written by others. Furthermore, when you start creating your own data structures, you’ll find it far easier to think about the overall flow of your program from the outset. It means you will essentially be able to organise your code and have a much clearer vision of how to go about writing each step of a program.
One of the core ideas in the collaborative work between Babbage and Lovelace, was a concept they called variables. A variable is a means for storing data. We can store numbers, text, images, boolean states, colours, objects and almost any kind of data type we define. We can access that data from anywhere in our program, use it and more importantly modify it. Indeed, the real interest in variables is being able to modify them over time during the running of the program. Variables give us variable state. In the world of dynamic graphics, this means we can change shape, form, colour, position and whatever else we decide to modify.
There are two main steps to creating a variable: 1). Declare. 2). Assign.
To declare a variable, we often need to declare it’s data type. More will be written about this in the next chapter, suffice to say that there exist a handful of primitive data types which tell the computer what kind of data we are going to store. With a language like Processing, we must be explicit about those data types. Whereas with javaScript and hence P5js, the language is less strict on this matter.
Along with the data type we must also give our variable a symbolic name. The name identifies the variable, the value of which is what we call an object, (more on the concept of objects later). The name we choose is entirely up to the programmer and it is usually chosen in accordance to it’s use. i.e. a variable’s name is logically chosen in line with what we intend it to be used for. For example, we may name a variable 'diameter' because we would like to store a numeric value for modifying the size of a circle. As you start to read more code, you’ll begin to notice a number of conventions for naming variables and it’s good practice to follow suit. Clear code should be made as human readable as possible. In general, nouns are used for variable names and you’ll see why later on.
The second step is to assign our variable with an initial value. This is a starting value which will change during the course of a program’s execution. It doesn't have to of course. This value could remain the same and this is what we call a constant. However, most variable values change during the running of a program. Which brings us to an essential part; updating the variable. We can apply simple operations such as adding, subtracting or multyplying to modify our variable values. It is also entirely possible to apply operations on multiple variables and indeed, as we move on, you'll see that variables are used to modify parameters, or what we call arguments, for more complex structures, like functions for example.
data type
‹variable name›=
‹variable value›
Eg 1. js. *
let
x =
0;
Eg 2. java
float
x =
0;
* We are declaring
& assigning our variables all on one line.
// here we are updating our variables
x = x + 2;
dia = dia + 0.5;
Details
The variable is an essential concept yet it takes a little time to understand the full workings for the beginner. One of the reasons for this, is that the student doesn’t immediately grasp the flow of data within a program. It can be difficult at first to trace where we update the value of a variable and also how we use that variable within a function for example. When we start to develop more complex programs, understanding what updates a variable is not always so explicit. For example, a simple operation like dia = dia + 1; is easily traced – the value of 1. However in dia = dia * speed; the value of speed is stored in another variable and we’d need to read a little more of the code to see where this variable is declared and understand eventually the values it produces.
There are slight variations in the manner we declare and eventually assign variables. For example, we can both declare and assign all on one line, as we have done above. However, it is equally possible to simply declare a variable in one part of our program and then assign it a value elsewhere. The best way to get accustomed to these conventions is to start reading simple programs oneself. In the next chapter, we'll look at primitive data types, the diffrence between strongly typed languages such as Processing and weakly typed ones such as JavaScript. We'll also look at a concept called variable scope within a program.
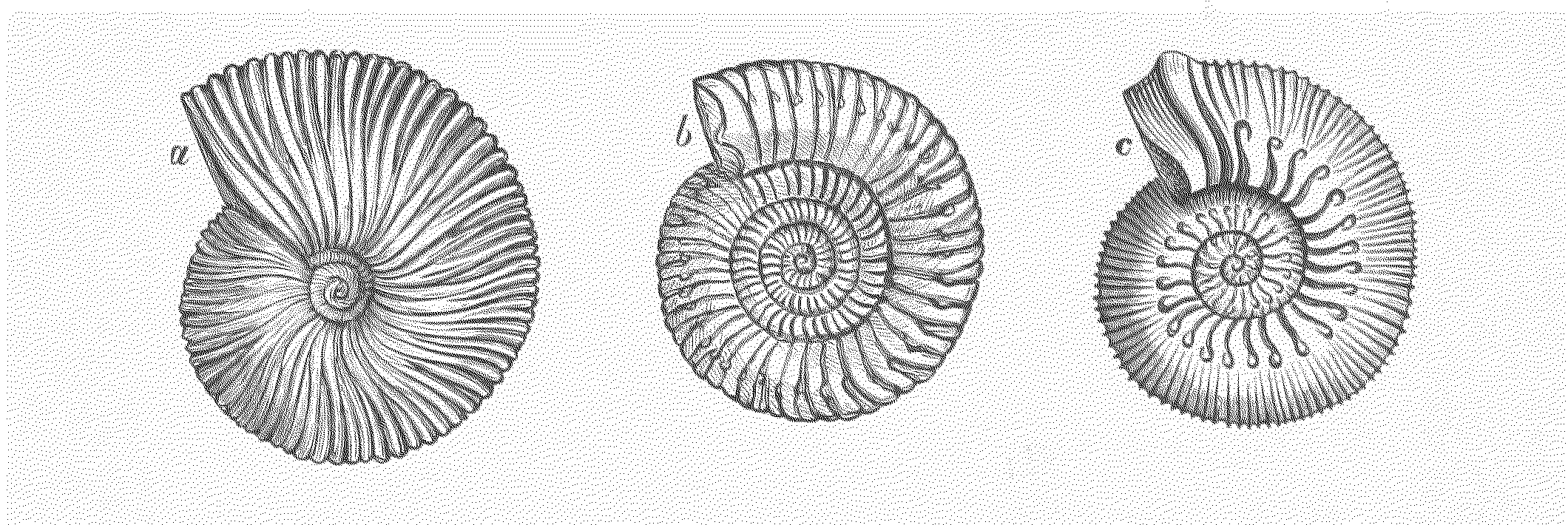
Variables observed in nature.Source: British Library